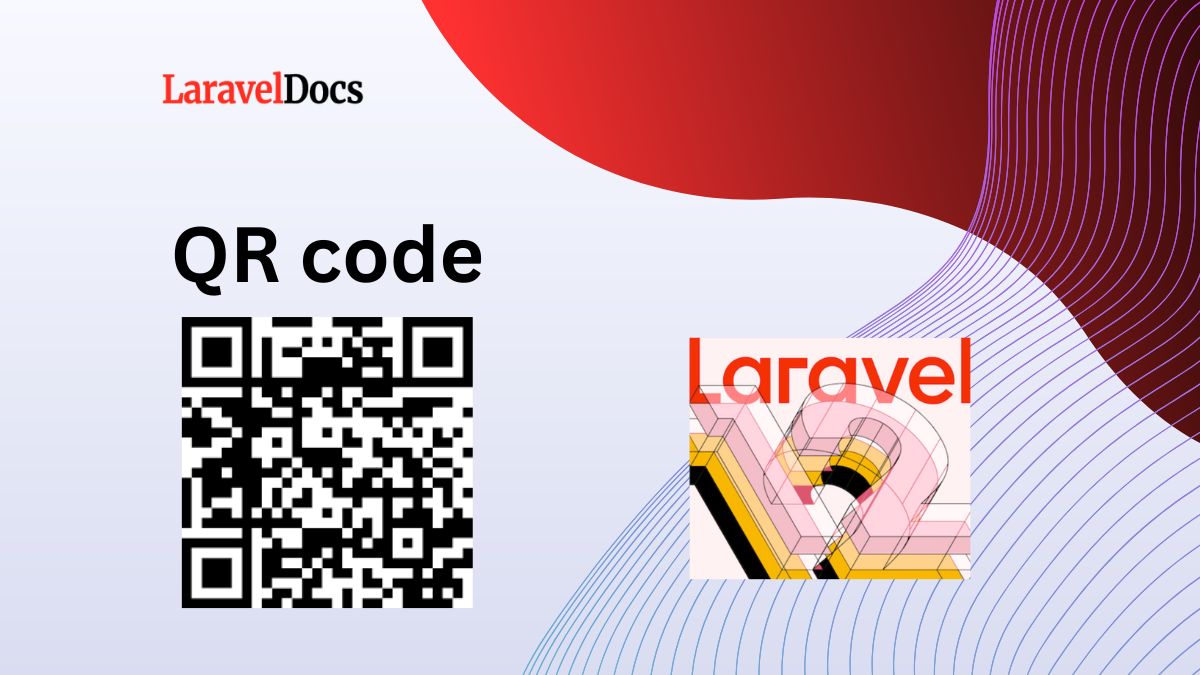
Laravel 12 Generate QR Code Example...
QR codes have become a widely used method for sharing information quickly and securely. From prod...
QR codes have become a widely used method for sharing information quickly and securely. From product labels to mobile payments, their versatility makes them a valuable tool in many applications. In this tutorial, we will explore how to generate QR codes in a Laravel 12 application using the simple-qrcode
package. This guide is beginner-friendly and will walk you through every step, from installing the package to rendering QR codes dynamically.
Before we begin, ensure you have the following:
Laravel 12 installed
Composer installed and configured
Basic understanding of Laravel controllers, routes, and views
Step 1: Install Laravel 12 App
If you not created Laravel app yet, install it with some simple steps.
Yoy may check our blog How to Create Laravel Project in your local machine.
composer global require laravel/installer laravel new laravel12-crud-app cd laravel12-crud-app npm install && npm run build composer run dev
Navigate to your project directory:
cd laravel12-crud-app
Run the development server:
php artisan serve
Laravel will be accessible at http://127.0.0.1:8000
.
To generate QR codes in Laravel, we will use the simple-qrcode
package, which is a Laravel wrapper around the popular library.
Run the following Composer command:
composer require simplesoftwareio/simple-qrcode
No additional configuration is necessary for Laravel 12, as package discovery is automatic.
Next, generate a controller that will handle the QR code logic.
php artisan make:controller QRCodeController
Open the newly created app/Http/Controllers/QRCodeController.php
file and add the following code:
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use SimpleSoftwareIO\QrCode\Facades\QrCode;
class QRCodeController extends Controller
{
public function index()
{
return view('qr-code');
}
public function generate(Request $request)
{
$data = $request->input('data');
$qrCode = QrCode::size(250)->generate($data);
return view('qr-code', compact('qrCode', 'data'));
}
}
Now, define the web routes that will point to our controller methods.
Open the routes/web.php
file and add:
use App\Http\Controllers\QRCodeController;
Route::get('/qr-code', [QRCodeController::class, 'index']);
Route::post('/qr-code', [QRCodeController::class, 'generate'])->name('qr-code.generate');
Create a new view file named qr-code.blade.php
in the resources/views
directory.
<!-- resources/views/qr-code.blade.php -->
<!DOCTYPE html>
<html>
<head>
<title>Laravel 12 QR Code Generator</title>
<meta charset="utf-8">
</head>
<body>
<h2>Laravel 12 Generate QR Code Example</h2>
<form action="{{ route('qr-code.generate') }}" method="POST">
@csrf
<label for="data">Enter Text or URL:</label>
<input type="text" name="data" required>
<button type="submit">Generate QR Code</button>
</form>
@if (!empty($qrCode))
<h3>QR Code for: {{ $data }}</h3>
<div>{!! $qrCode !!}</div>
@endif
</body>
</html>
This simple view includes a form to input data and a section to display the generated QR code.
Now, serve your Laravel application:
php artisan serve
Visit http://127.0.0.1:8000/qr-code
in your browser.
Enter a text string or URL into the form.
Submit the form.
The page will refresh and display a QR code corresponding to your input.
The package offers many customization options. Here are some examples:
php
Copy
QrCode::size(400) ->color(255, 0, 0) ->generate('Custom colored QR code');
php
Copy
QrCode::size(300) ->backgroundColor(255, 255, 0) ->generate('QR code with yellow background');
php
Copy
// PNG format QrCode::format('png')->generate('PNG QR code'); // SVG format (default) QrCode::format('svg')->generate('SVG QR code'); // EPS format QrCode::format('eps')->generate('EPS QR code');
php
Copy
QrCode::email('test@example.com', 'Test Subject', 'This is the message body');
php
Copy
QrCode::phoneNumber('555-555-5555');
php
Copy
QrCode::SMS('555-555-5555', 'This is the SMS message body');
php
Copy
QrCode::wiFi([ 'encryption' => 'WPA', 'ssid' => 'Network Name', 'password' => 'Network Password', 'hidden' => 'Whether the network is a hidden SSID or not.' ]);
You can create a QR code with a logo in the center:
php
Copy
QrCode::format('png') ->size(300) ->merge(public_path('images/logo.png'), 0.3, true) ->generate('QR code with logo');
The merge()
method parameters are:
Path to the logo image
Percentage of the QR code size the logo should occupy (0.3 = 30%)
Boolean indicating whether to maintain the logo's aspect ratio
QR codes have error correction capabilities. You can set the level:
php
Copy
QrCode::errorCorrection('H')->generate('QR code with high error correction');
Available levels:
L - Low (7% of codewords can be restored)
M - Medium (15% can be restored)
Q - Quartile (25% can be restored)
H - High (30% can be restored)
You can customize the style of the QR code:
php
Copy
QrCode::style('dot') ->eye('circle') ->color(0, 0, 255) ->generate('Styled QR code');
Available styles:
square
(default)
dot
round
Available eye styles:
square
(default)
circle
You might want to return QR codes from your API. Here's an example:
php
Copy
Route::get('/api/qrcode', function () { $qrCode = QrCode::size(200)->generate('API QR Code'); return response()->json([ 'qr_code' => $qrCode, 'message' => 'Scan this QR code' ]); });
For binary responses (like PNG images):
php
Copy
Route::get('/api/qrcode-png', function () { $image = QrCode::format('png')->size(200)->generate('PNG QR Code from API'); return response($image)->header('Content-type', 'image/png'); });
1. Size Matters: Ensure your QR code is large enough to be easily scanned (minimum 2cm x 2cm for print, 100px x 100px for digital).
2. Contrast: Always use high contrast between the QR code and background (typically black on white).
3. Content Length: The more data you encode, the denser (and potentially harder to scan) the QR code becomes.
4. Testing: Always test your QR codes with multiple devices and scanning apps.
5. Error Correction: Use higher error correction (H or Q) if the QR code might be damaged or obscured.
If you want to save the generated QR code as an image file, modify the generate()
method as follows:
public function generate(Request $request)
{
$data = $request->input('data');
$image = \QrCode::format('png')->size(250)->generate($data);
$outputFile = '/qr-code-' . time() . '.png';
\Storage::disk('public')->put($outputFile, $image);
$imageUrl = asset('storage' . $outputFile);
return view('qr-code', compact('imageUrl', 'data'));
}
Then, update the Blade view to include:
@if (!empty($imageUrl))
<h3>QR Code for: {{ $data }}</h3>
<img src="{{ $imageUrl }}" alt="QR Code">
@endif
Make sure you have a symbolic link to the storage directory:
php artisan storage:link
In this tutorial, we demonstrated how to generate QR codes using Laravel 12 and the simple-qrcode
package. You learned how to create a controller, define routes, build a simple form, and display QR codes dynamically based on user input. You also saw how to optionally save the QR code as an image for download or storage.
With just a few lines of code, you can now integrate QR code functionality into your Laravel applications—whether for product tagging, event tickets, or secure links.
GitHub repository:
With over a decade of experience in web development, I have always been a huge lover of PHP, Laravel, Wordpress Development, other CMS, Javascript, Bootstrap, Tailwind CSS and Alpine JS. I am passionate about sharing my knowledge and experience with others, and I believe that learning is a lifelong journey.
QR codes have become a widely used method for sharing information quickly and securely. From prod...
Introduction
Laravel 12 continues to be a robust, elegant PHP framewor...
If you're aiming to become a Laravel developer or improve your Laravel skills, you're in...
Subscribe to my newsletter to get updated posts