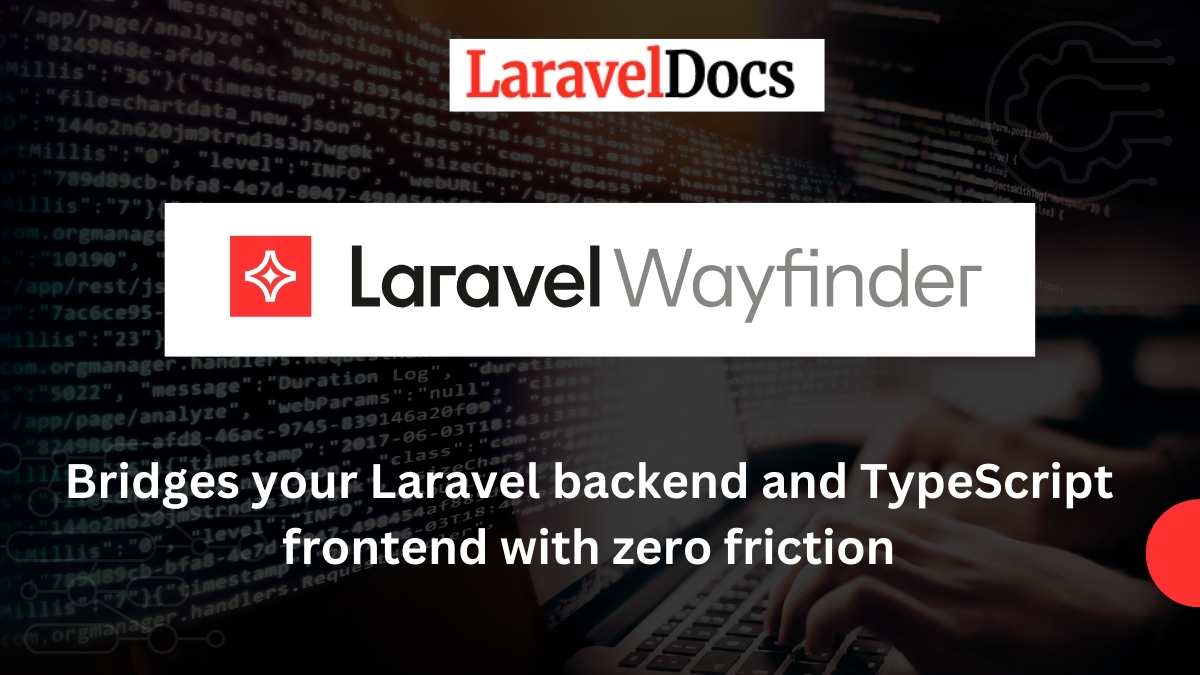
Laravel Wayfinder | Bridging Larave...
One new innovative tool of Laravel is Laravel Wayfinder, a package designed to seamlessly connect...
One new innovative tool of Laravel is Laravel Wayfinder, a package designed to seamlessly connect Laravel's PHP backend with TypeScript frontends. In the ever-evolving landscape of web development, integrating backend and frontend technologies efficiently remains a pivotal challenge. Laravel, renowned for its elegant syntax and robust features, has continually addressed these challenges by introducing tools that streamline development processes.
This article delves deep into Laravel Wayfinder, exploring its features, installation, usage, and the myriad benefits it offers to developers.
As web applications grow in complexity, the need for a harmonious integration between the server-side logic and client-side scripts becomes increasingly critical. Traditionally, developers have grappled with hardcoding URLs, manually synchronizing route parameters, and ensuring consistency between backend routes and frontend calls. These tasks are not only tedious but also prone to errors, leading to potential maintenance nightmares.
Laravel Wayfinder emerges as a solution to these challenges. By automatically generating fully-typed, importable TypeScript functions for Laravel controllers and routes, Wayfinder eliminates the need for manual synchronization. This automation ensures that frontend applications can invoke backend endpoints as if they were native functions, thereby enhancing developer productivity and reducing the likelihood of errors.
Automatic TypeScript Function Generation: Wayfinder scans Laravel controllers and routes to generate corresponding TypeScript functions. This automation ensures that any changes in the backend are immediately reflected in the frontend, maintaining consistency across the application.
Fully-Typed Functions: The generated TypeScript functions come with complete type definitions, leveraging TypeScript's static typing capabilities. This feature enhances code reliability by catching type-related errors during development rather than at runtime.
Seamless Integration with Vite: Wayfinder integrates smoothly with Vite, a modern frontend build tool. This integration facilitates efficient hot module replacement and rapid development cycles.
Customizable Generation Paths: Developers can specify the directories where the TypeScript definitions should be generated, allowing for flexibility in project structure.
Support for Various HTTP Methods: The generated functions support multiple HTTP methods, enabling developers to perform GET, POST, PUT, DELETE, and other requests effortlessly.
Handling of Reserved JavaScript Keywords: Wayfinder intelligently renames functions that might conflict with JavaScript reserved keywords, ensuring that the generated code remains valid and functional.
Integrating Laravel Wayfinder into a project is straightforward. The following steps outline the installation and configuration process:
Begin by adding Wayfinder to your Laravel project using Composer:
composer require laravel/wayfinder
For automatic detection of changes in routes and controllers, install the vite-plugin-run
npm package:
npm install -D vite-plugin-run
Update your vite.config.js
file to watch for changes in your application's routes and controllers:
import { defineConfig } from 'vite';
import { run } from 'vite-plugin-run';
export default defineConfig({
plugins: [
// Other plugins...
run([
{
name: 'wayfinder',
run: ['php', 'artisan', 'wayfinder:generate'],
pattern: ['routes/**/*.php', 'app/**/Http/**/*.php'],
},
]),
],
resolve: {
alias: {
'@actions/': './resources/js/actions',
'@routes/': './resources/js/routes',
},
},
});
This configuration ensures that any changes to routes or controllers trigger the regeneration of TypeScript definitions, keeping the frontend in sync with the backend.
Manually generate the TypeScript definitions by running:
php artisan wayfinder:generate
For continuous synchronization during development, the Vite plugin will handle automatic regeneration.
Once Wayfinder is set up, you can leverage the generated TypeScript functions in your frontend code. Here's how:
Import the desired function from the generated files:
import { show } from '@actions/App/Http/Controllers/PostController';
Invoke the function with the necessary parameters:
const { url, method } = show(1);
Alternatively, if only the URL is needed:
const postUrl = show.url(1);
For different HTTP methods:
const { url, method } = show.head(1);
Wayfinder functions are versatile in handling parameters:
Single Parameter Actions:
show(1);
show({ id: 1 });
Multiple Parameter Actions:
update([1, 2]);
update({ post: 1, author: 2 });
update({ post: { id: 1 }, author: { id: 2 } });
This flexibility ensures that the functions can accommodate various data structures and parameter passing conventions.
If you wish to skip the generation of action or route definitions, use the following commands:
Skip Actions:
php artisan wayfinder:generate --skip-actions
Skip Routes:
php artisan wayfinder:generate --skip-routes
By default, Wayfinder generates files in the resources/js/wayfinder
, resources/js/actions
, and resources/js/routes
directories. To customize the base path:
php artisan wayfinder:generate --path=resources/js/custom-path
In cases where controller methods have names that are reserved JavaScript keywords (e.g., delete
, import
), Wayfinder appends the word "Method" to the function name to avoid conflicts:
import { deleteMethod } from '@actions/App/Http/Controllers/PostController';
Enhanced Developer Productivity: By automating the generation of TypeScript functions, developers can focus more on building features rather than managing boilerplate code.
Consistency Across Backend and Frontend: Automatic synchronization ensures that any changes in the backend are immediately available in the frontend, reducing the risk of mismatches and errors.
Type Safety: Leveraging TypeScript's static typing, Wayfinder helps catch potential errors during development, leading to more robust and reliable applications.
Simplified Maintenance: With a clear and consistent interface between the backend and frontend, maintaining and updating the application becomes more straightforward.
Flexibility: Customizable paths and the ability to handle various parameter structures provide developers with the flexibility to tailor Wayfinder to their specific project needs.
Let’s now look at some practical use cases, potential pitfalls, and community adoption insights to round out your understanding.
Modern SPAs built using Vue, React, or even Svelte rely heavily on API endpoints. Traditionally, frontend developers must manually write endpoints like:
fetch(`/api/posts/${id}`, { method: 'GET' });
If the route changes in Laravel, the frontend breaks unless manually updated. With Wayfinder, this becomes:
import { show } from '@actions/App/Http/Controllers/PostController';
const { url, method } = show(5);
fetch(url, { method });
When the backend changes (e.g., URL changes from /posts/{id}
to /articles/{id}
), Wayfinder regenerates the correct URLs and methods, making frontend maintenance almost effortless.
Complex resource routes that take multiple parameters—like nested resources—are a breeze with Wayfinder. Suppose you have a route like:
Route::get('/authors/{author}/posts/{post}', [PostController::class, 'show']);
With Wayfinder, you’d simply call:
show({ author: 1, post: 12 });
No need to guess parameter ordering or worry about missing required inputs—everything is inferred and typed.
When building dynamic forms where the form's action
attribute or JavaScript handler relies on backend routes, Wayfinder is extremely helpful.
<form action={update.url(postId)} method="POST">
Or, for JavaScript form submissions:
const { url, method } = update(postId);
await fetch(url, {
method,
body: JSON.stringify(payload),
headers: {
'Content-Type': 'application/json',
},
});
In cases where Blade templates are still being used alongside modern JavaScript tooling (e.g., Alpine.js + Vite + TypeScript), Wayfinder still adds value. You can share route logic between your Blade templates and the frontend via shared JS components that use Wayfinder-generated paths.
While Wayfinder streamlines a lot of your Laravel + frontend development workflow, there are a few things to be aware of:
If you're not already using TypeScript, Wayfinder might feel unfamiliar at first. Understanding generics, typings, and how routes translate into functions requires a TypeScript-first mindset.
Wayfinder assumes your Laravel routes are defined using conventional controller methods (index
, show
, store
, etc.). Custom actions or closures may not generate as expected unless explicitly structured.
If you’re working in a very large application, automatic regeneration on every file change (using Vite) can feel heavy. It’s advisable to optimize when and how often Wayfinder runs in development environments.
Wayfinder shines with controller-based routing. If your app uses many route closures or is heavily customized, you may not get full value out of the package unless you restructure some routing logic.
Laravel Wayfinder is still relatively new but has already gained attention in the Laravel community due to its seamless dev experience. Created by Laravel community member tnylea, it follows Laravel’s design philosophy—expressive, elegant syntax with developer experience at the core.
Community conversations around Wayfinder often highlight how well it works with:
Inertia.js — Making full-stack JS/Laravel hybrid apps feel native.
Vue + TypeScript projects — Especially those bootstrapped with Laravel Breeze or Jetstream.
Monorepos — Where you have separate backend and frontend apps but need them to speak the same language.
The Laravel ecosystem has always embraced productivity and developer happiness. With Laravel Wayfinder, we’re witnessing the next step in full-stack Laravel development—one where backend logic can be consumed seamlessly in the frontend.
Here’s what we might see in the future:
Automatic FormRequest integration: Generating validation rules/types based on Laravel’s FormRequest classes.
Better IntelliSense in VSCode: Autocompletion across IDEs with more rich data for routes and parameters.
Support for route middleware insights: Understanding auth guards, rate limits, and other route metadata in the frontend.
Dynamic API Documentation: Leveraging Wayfinder’s route metadata to generate OpenAPI docs or frontend route explorers.
Laravel Wayfinder represents a significant advancement in bridging the gap between Laravel's backend and TypeScript-based frontends. By automating the generation of fully-typed, importable TypeScript functions for controllers and routes, it streamlines the development process, enhances code reliability, and ensures consistency across the application. As web development continues to evolve, tools like Wayfinder play a crucial role in enabling developers to build more efficient, maintainable, and scalable applications.
Whether you're working on a single-page application (SPA), integrating with Inertia.js, or building modern Vue or React components in a Laravel environment, Laravel Wayfinder brings a layer of abstraction that reduces the mental load of managing API routes manually.
Laravel Wayfinder is not just a convenience—it’s a paradigm shift in how frontend and backend developers collaborate in Laravel applications. By providing a bridge between controller routes and TypeScript-powered frontends, it solves a long-standing pain point: keeping routes, methods, and parameters in sync.
Whether you're working solo or on a large team, Wayfinder reduces cognitive load, cuts down on bugs, and improves the maintainability of your project.
If you're building a modern Laravel app with a TypeScript frontend, Wayfinder is no longer just a nice-to-have—it’s a must.
Laravel Wayfinder generates TypeScript functions from Laravel controllers/routes.
Fully typed and importable functions make it easy to call Laravel routes in the frontend.
Great for SPAs, Vue/React apps, and TypeScript-first projects.
Seamless integration with Vite.
Reduces route-related bugs, increases dev confidence.
Still evolving, but already powerful and production-ready.
With over a decade of experience in web development, I have always been a huge lover of PHP, Laravel, Wordpress Development, other CMS, Javascript, Bootstrap, Tailwind CSS and Alpine JS. I am passionate about sharing my knowledge and experience with others, and I believe that learning is a lifelong journey.
One new innovative tool of Laravel is Laravel Wayfinder, a package designed to seamlessly connect...
Subscribe to my newsletter to get updated posts