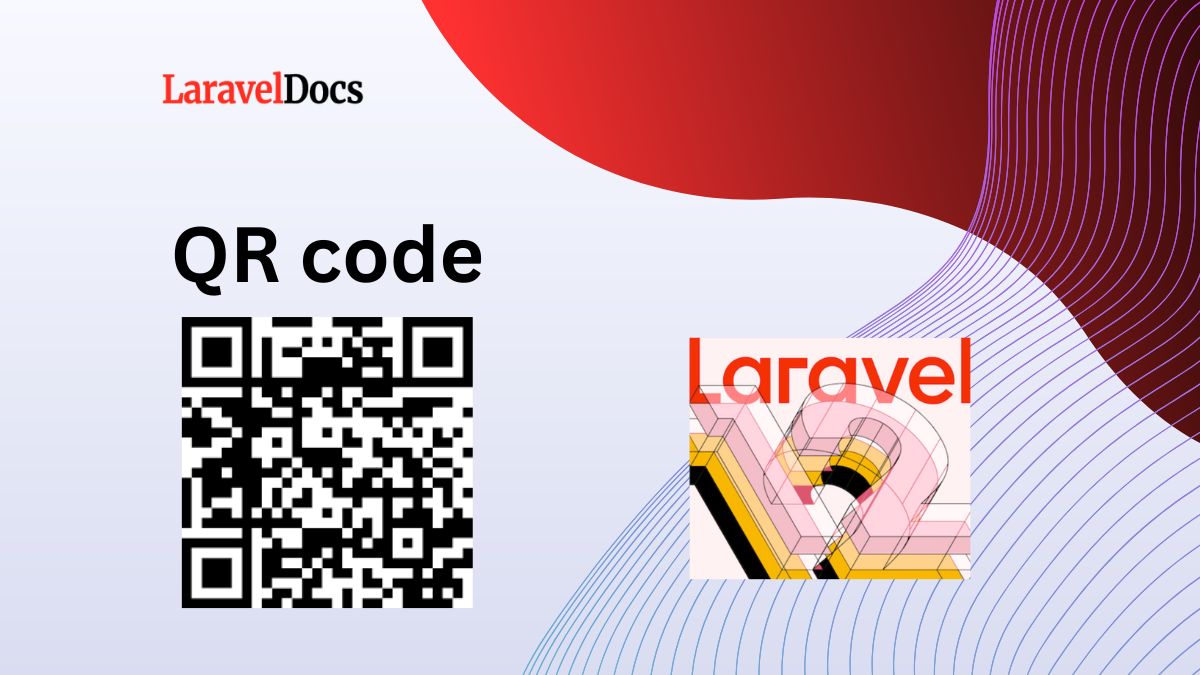
Laravel 12 Generate QR Code Example...
QR codes have become a widely used method for sharing information quickly and securely. From prod...
Introduction
Laravel 12 continues to be a robust, elegant PHP framework that simplifies web development. One of the most common functionalities in any web application is CRUD: Create, Read, Update, and Delete. This tutorial is designed for beginners who want to understand and implement a basic CRUD operation in Laravel 12. By the end of this guide, you will have a working application to manage records in a MySQL database.
Prerequisites
Before you begin, ensure that you have the following installed:
PHP >= 8.2
Composer
Laravel Installer
MySQL or any other supported database
Code editor like Visual Studio Code
Step 1: Install Laravel 12 App
If you not created Laravel app yet, install it with some simple steps.
Yoy may check our blog How to Create Laravel Project in your local machine.
composer global require laravel/installer laravel new laravel12-crud-app cd laravel12-crud-app npm install && npm run build composer run dev
Navigate to your project directory:
cd laravel12-crud-app
Run the development server:
php artisan serve
Laravel will be accessible at http://127.0.0.1:8000
.
Step 2: Creating Model, Migration, and Controller
Let us assume we are building a CRUD for managing "Posts". Run the following Artisan command:
php artisan make:model Post -mc
This command creates a model, migration file, and controller.
Step 3: Database Setup and Migration
Open the .env
file and update the database credentials:
DB_DATABASE=your_database_name
DB_USERNAME=your_username
DB_PASSWORD=your_password
Now, open the migration file located in database/migrations/
and define the schema:
Schema::create('posts', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->text('content');
$table->timestamps();
});
Run the migration:
php artisan migrate
Step 4: Building Routes and Controllers
Define resource routes in routes/web.php
:
use App\Http\Controllers\PostController;
Route::resource('posts', PostController::class);
Now, open the PostController.php
and define the methods for handling CRUD:
public function index() {
$posts = Post::all();
return view('posts.index', compact('posts'));
}
public function create() {
return view('posts.create');
}
public function store(Request $request) {
$request->validate([
'title' => 'required',
'content' => 'required',
]);
Post::create($request->all());
return redirect()->route('posts.index')
->with('success', 'Post created successfully.');
}
public function show(Post $post) {
return view('posts.show', compact('post'));
}
public function edit(Post $post) {
return view('posts.edit', compact('post'));
}
public function update(Request $request, Post $post) {
$request->validate([
'title' => 'required',
'content' => 'required',
]);
$post->update($request->all());
return redirect()->route('posts.index')
->with('success', 'Post updated successfully.');
}
public function destroy(Post $post) {
$post->delete();
return redirect()->route('posts.index')
->with('success', 'Post deleted successfully.');
}
Step 5: Designing Blade Views
Create the views inside resources/views/posts/
. You need the following files: index.blade.php
, create.blade.php
, edit.blade.php
, and show.blade.php
.
Example: index.blade.php
@extends('layouts.app')
@section('content')
<h1>Posts</h1>
<a href="{{ route('posts.create') }}">Create New Post</a>
@if ($message = Session::get('success'))
<p>{{ $message }}</p>
@endif
<table>
<thead>
<tr>
<th>Title</th>
<th>Content</th>
<th>Actions</th>
</tr>
</thead>
<tbody>
@foreach ($posts as $post)
<tr>
<td>{{ $post->title }}</td>
<td>{{ $post->content }}</td>
<td>
<a href="{{ route('posts.show', $post->id) }}">View</a>
<a href="{{ route('posts.edit', $post->id) }}">Edit</a>
<form action="{{ route('posts.destroy', $post->id) }}" method="POST">
@csrf
@method('DELETE')
<button type="submit">Delete</button>
</form>
</td>
</tr>
@endforeach
</tbody>
</table>
@endsection
Repeat this pattern for create
, edit
, and show
views.
Step 6: Form Validation and Error Handling
Use Laravel’s built-in validation to display errors. In your form views, you can add:
@if ($errors->any())
<ul>
@foreach ($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
@endif
This will show validation errors above the form.
Step 7: Testing the CRUD Operations
Run your application using php artisan serve
and test each of the CRUD operations:
Create a new post
View the list of posts
Edit a post
Delete a post
Ensure each operation updates the database correctly.
Step 8: Deploying the Application
Once your application is ready, you can deploy it using:
Shared hosting (via cPanel)
Laravel Forge
Heroku (with Procfile and database setup)
Upload your project files, configure the .env
file, and run php artisan migrate --force
to apply migrations.
Conclusion
Congratulations! You’ve successfully built a simple CRUD application using Laravel 12. This tutorial introduced you to the foundational concepts of Laravel, including routing, controllers, models, and Blade templating. As a next step, consider adding features like authentication, search, or pagination to enhance your application further.
Stay tuned for more Laravel tutorials and best practices to take your development skills to the next level.
With over a decade of experience in web development, I have always been a huge lover of PHP, Laravel, Wordpress Development, other CMS, Javascript, Bootstrap, Tailwind CSS and Alpine JS. I am passionate about sharing my knowledge and experience with others, and I believe that learning is a lifelong journey.
QR codes have become a widely used method for sharing information quickly and securely. From prod...
Introduction
Laravel 12 continues to be a robust, elegant PHP framewor...
If you're aiming to become a Laravel developer or improve your Laravel skills, you're in...
Subscribe to my newsletter to get updated posts