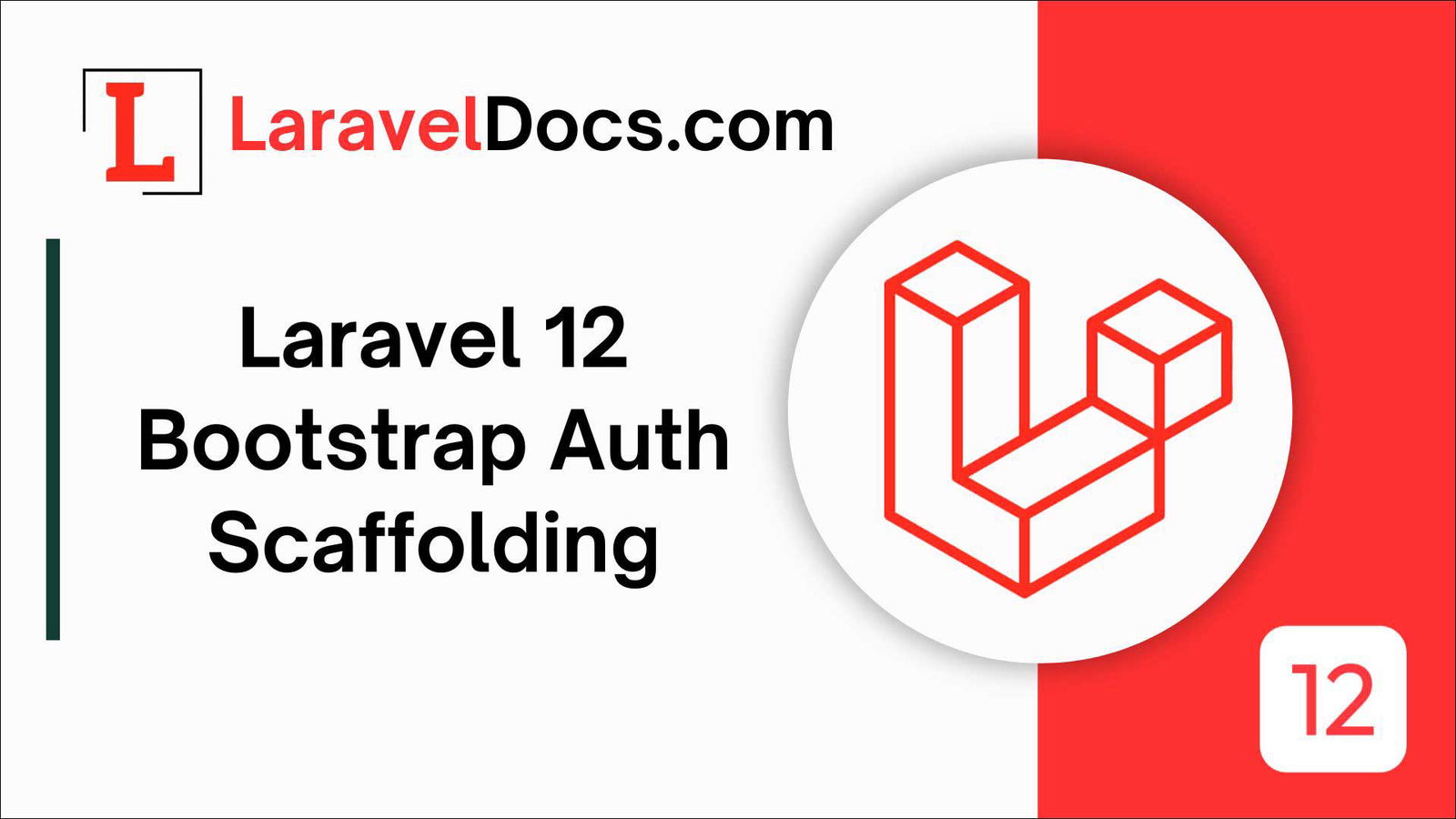
Laravel 12 Bootstrap Auth Scaffoldi...
Laravel 12 brings enhanced features, security improvements, and flexibility for developers. If yo...
Laravel 12 brings enhanced features, security improvements, and flexibility for developers. If you want to set up a Bootstrap-based authentication system quickly, Laravel provides built-in authentication scaffolding that makes it easier than ever.
In this tutorial, we will walk through the process of setting up authentication using Bootstrap in Laravel 12.
Before getting started, ensure that your system has PHP 8+, Composer, and a database (MySQL, PostgreSQL, SQLite, etc.) installed.
Run the following command to create a new Laravel 12 project:
composer global require laravel/installer
Then run the following command:
laravel new laravel12-auth
Once the installation is complete, navigate to the project directory:
cd laravel12-auth
Update the .env
file with your database credentials:
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=laravel12_auth
DB_USERNAME=root
DB_PASSWORD=
Run database migrations:
php artisan migrate
This will create the necessary authentication tables.
Laravel provides an official package called laravel/ui
to generate authentication scaffolding. Install it using Composer:
composer require laravel/ui
Now, install the Bootstrap authentication scaffolding:
php artisan ui bootstrap --auth
This command will generate authentication views, controllers, and routes.
To install Node.js dependencies, run:
npm install && npm run dev
This will compile the frontend assets, including Bootstrap styles.
The authentication UI is already set up, but you can customize it by modifying the layout file located at:
resources/views/layouts/app.blade.php
For example, to add a navbar, update it like this:
Start the local development server:
php artisan serve
Now, visit http://127.0.0.1:8000/login
or http://127.0.0.1:8000/register
to see the authentication system in action.
Then, create a new dashboard route in routes/web.php
:
Route::get('/dashboard', function () {
return view('dashboard');
})->middleware(['auth']);
Create a new dashboard.blade.php
file in resources/views/
:
@extends('layouts.app')
@section('content')
<div class="container">
<div class="row justify-content-center">
<div class="col-md-8">
<div class="card">
<div class="card-header">Dashboard</div>
<div class="card-body">
You are logged in!
</div>
</div>
</div>
</div>
</div>
@endsection
In this tutorial, we successfully set up Laravel 12 Bootstrap Authentication Scaffolding in just a few steps. You now have a fully functional login, registration, and logout system with Bootstrap styling.
[media url="https://www.youtube.com/embed/_5juSaCrJv4"][/media]
resources/views/auth/
)
Stay curious, keep coding!
Ethan specializes in securing Laravel applications, providing insights into authentication, authorization, data protection, and vulnerability prevention. With over 10 years of cybersecurity experience, Ethan helps developers write secure, robust code.
Laravel 12 brings enhanced features, security improvements, and flexibility for developers. If yo...
Subscribe to my newsletter to get updated posts