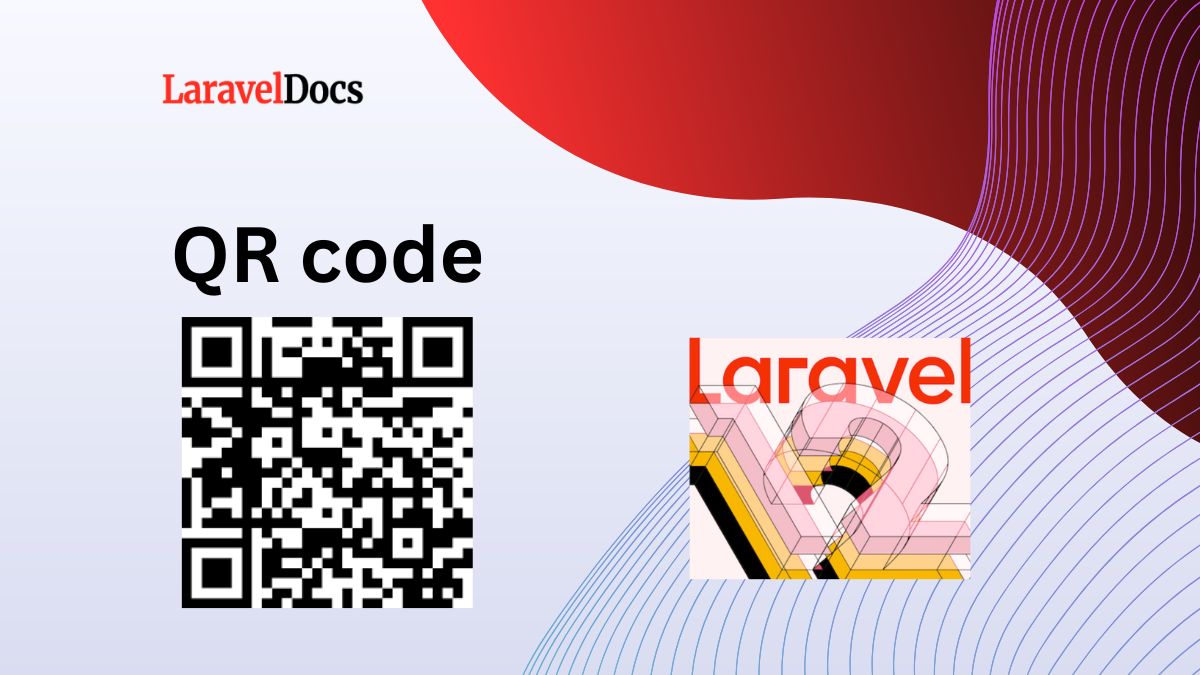
Laravel 12 Generate QR Code Example...
QR codes have become a widely used method for sharing information quickly and securely. From prod...
When you are new to Laravel, understanding how your application processes a request might feel like a mystery. But don’t worry—Laravel's architecture is beautifully designed and knowing the request lifecycle can empower you to write cleaner, more efficient code.
Laravel is one of the most popular PHP frameworks, known for its elegance, simplicity, and powerful features. If you're just starting with Laravel 12, understanding how a request moves through the application is crucial. This process is known as the request lifecycle. In this blog, we’ll break down the Laravel 12 request lifecycle into simple, easy-to-understand steps. By the end, you’ll have a clear picture of how Laravel handles an HTTP request and generates a response.
The request lifecycle in Laravel refers to the journey an HTTP request takes from the moment it enters the application until a response is sent back to the client. Laravel’s lifecycle is designed to be modular and extensible, allowing developers to customize and extend its behavior at various stages.
Let’s dive into the key phases of the Laravel 12 request lifecycle:
Every Laravel application starts with an HTTP request. This could be a GET request to load a webpage, a POST request to submit a form, or any other type of HTTP request.
The request is first received by the public/index.php file, which is the entry point for all requests in a Laravel application.
This file loads the Composer autoloader and bootstraps the Laravel application.
// public/index.php (Laravel 12)
use Illuminate\Foundation\Application;
use Illuminate\Http\Kernel;
use Illuminate\Http\Request;
require __DIR__ . '/../vendor/autoload.php';
$app = Application::configure()
->withRouting(
web: __DIR__ . '/../routes/web.php',
api: __DIR__ . '/../routes/api.php',
commands: __DIR__ . '/../routes/console.php',
channels: __DIR__ . '/../routes/channels.php',
)
->withMiddleware(function () {
return require __DIR__ . '/../app/Http/middleware.php';
})
->create();
$app->make(Kernel::class)
->handle(Request::capture())
->send();
This file boots up the Laravel framework, loads dependencies via Composer, and captures the incoming request.
Once the request enters the application, Laravel begins the bootstrapping process. This involves:
Loading environment variables from the .env
file.
Registering service providers defined in the config/app.php
file.
Bootstrapping essential components like the database, cache, and session.
The bootstrapping process ensures that the application is ready to handle the incoming request.
Middleware are filters that can inspect and modify the HTTP request before it reaches your application logic and again before the response is sent back.
Examples:
Checking if the user is authenticated.
Validating CSRF tokens.
Logging request data.
Laravel has global middleware (applies to all requests) and route-specific middleware (applies to specific routes).
Laravel then boots up all service providers listed in config/app.php
. These are the central place to configure application components, such as:
Database connections
Event listeners
Custom services
This step ensures your app is ready to handle logic.
Once middleware is applied, Laravel uses its powerful routing system to match the request URL and HTTP verb (GET, POST, etc.) to a defined route.
Routes are typically defined in:
routes/web.php
for web routes
routes/api.php
for API routes
Each route points to a controller or a closure that handles the business logic.
If your route is pointing to a controller, the request now reaches that controller method. This is where you:
Fetch data from the database
Apply business logic
Call services or helpers
Finally, the controller returns a response (HTML, JSON, redirect, etc.) back to the user.
Finally, the response is sent back to the client. This could be an HTML page, a JSON object, or a redirect.
Laravel uses the Symfony Response
class to handle HTTP responses.
The response is sent to the browser or client, completing the request lifecycle.
User Request
↓
public/index.php
↓
vendor/autoload.php
↓
bootstrap/app.php
↓
bootstrap/providers.php
↓
Middleware (Pre-Processing)
↓
Routing (web.php/api.php)
↓
Controller/Closure
↓
Response Created
↓
Middleware (Post-Processing)
↓
Response Sent to Browser
The Laravel request lifecycle is a structured process that ensures every request is handled efficiently.
Middleware plays a crucial role in filtering requests and modifying responses.
Understanding the lifecycle helps you debug issues, optimize performance, and extend Laravel’s functionality.
Explore Middleware: Middleware is a powerful feature in Laravel. Try creating custom middleware to see how it affects the request lifecycle.
Use Debugging Tools: Tools like Laravel Telescope can help you visualize and debug the request lifecycle.
Read the Documentation: Laravel’s official documentation is an excellent resource for understanding advanced concepts.
Understanding Laravel’s request lifecycle helps you build more robust and efficient applications. From the moment a user clicks a link to the moment a response appears in their browser,
The Laravel 12 request lifecycle is a fundamental concept that every developer should understand. By breaking it down into simple steps, we’ve seen how a request moves through the application, from the initial HTTP request to the final response. Whether you’re building a simple blog or a complex web application, understanding this process will help you write better, more efficient code.
Start exploring each of these stages in your projects. Over time, you’ll develop a deeper understanding of Laravel's internals and become a more proficient Laravel developer.
With over a decade of experience in web development, I have always been a huge lover of PHP, Laravel, Wordpress Development, other CMS, Javascript, Bootstrap, Tailwind CSS and Alpine JS. I am passionate about sharing my knowledge and experience with others, and I believe that learning is a lifelong journey.
QR codes have become a widely used method for sharing information quickly and securely. From prod...
Introduction
Laravel 12 continues to be a robust, elegant PHP framewor...
If you're aiming to become a Laravel developer or improve your Laravel skills, you're in...
Subscribe to my newsletter to get updated posts